MessengerContext
- Message Content Types
- Templates
- Quick Replies
- Sender Actions
- Targeting Broadcast Messages
- User Profile API
- Handover Protocol API
sendMessage(message [, options])
Send messages to the user using the Send API.
Param | Type | Description |
---|---|---|
message | Object | message object. |
options | Object | Other optional parameters. For example, messaging types or tags. |
Example:
context.sendMessage({
text: 'Hello!',
});
You can specify messaging type using options. If messagingType
and tag
is not provided, UPDATE
will be used as default messaging type.
Example:
context.sendMessage(
{
text: 'Hello!',
},
{
messagingType: 'RESPONSE',
}
);
Available messaging types:
UPDATE
as defaultRESPONSE
using{ messagingType: 'RESPONSE' }
optionsMESSAGE_TAG
using{ tag: 'ANY_TAG' }
options
Message Content Types - Official Docs
sendText(text [, options])
Send plain text messages to the user using the Send API.
Param | Type | Description |
---|---|---|
text | String | Text of the message to be sent. |
options | Object | Other optional parameters. For example, messaging types or tags. |
Example:
context.sendText('Hello!');
With CONFIRMED_EVENT_UPDATE
tag:
context.sendText('Hello!', { tag: 'CONFIRMED_EVENT_UPDATE' });
sendAttachment(attachment [, options])
Send attachment messages to the user using the Send API.
Param | Type | Description |
---|---|---|
attachment | Object | attachment object. |
options | Object | Other optional parameters. For example, messaging types or tags. |
Example:
context.sendAttachment({
type: 'image',
payload: {
url: 'https://example.com/pic.png',
},
});
sendAudio(audio [, options])
Send sounds to the user by uploading them or sharing a URL using the Send API.
Param | Type | Description |
---|---|---|
audio | String | Buffer | ReadStream | AttachmentPayload | The audio to be sent. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
- Send audio using URL string:
context.sendAudio('https://example.com/audio.mp3');
- Use
AttachmentPayload
to send cached attachment:
context.sendAudio({ attachmentId: '55688' });
- Use
ReadStream
created from local file:
const fs = require('fs');
context.sendAudio(fs.createReadStream('audio.mp3'));
sendImage(image [, options])
Send images to the user by uploading them or sharing a URL using the Send API. Supported formats are jpg, png and gif.
Param | Type | Description |
---|---|---|
image | String | Buffer | ReadStream | AttachmentPayload | The image to be sent. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
- Send image using URL string:
context.sendImage('https://example.com/vr.jpg');
- Use
AttachmentPayload
to send cached attachment:
context.sendImage({ attachmentId: '55688' });
- Use
ReadStream
created from local file:
const fs = require('fs');
context.sendImage(fs.createReadStream('vr.jpg'));
sendVideo(video [, options])
Send videos to the user by uploading them or sharing a URL using the Send API.
Param | Type | Description |
---|---|---|
video | String | Buffer | ReadStream | AttachmentPayload | The video to be sent. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
- Send video using URL string:
context.sendVideo('https://example.com/video.mp4');
- Use
AttachmentPayload
to send cached attachment:
context.sendVideo({ attachmentId: '55688' });
- Use
ReadStream
created from local file:
const fs = require('fs');
context.sendVideo(fs.createReadStream('video.mp4'));
sendFile(file [, options])
Send files to the user by uploading them or sharing a URL using the Send API.
Param | Type | Description |
---|---|---|
file | String | Buffer | ReadStream | AttachmentPayload | The file to be sent. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
- Send file using URL string:
context.sendFile('https://example.com/receipt.pdf');
- Use
AttachmentPayload
to send cached attachment:
context.sendFile({ attachmentId: '55688' });
- Use
ReadStream
created from local file:
const fs = require('fs');
context.sendFile(fs.createReadStream('receipt.pdf'));
Templates - Official Docs
sendTemplate(template [, options])
Send structured message templates to the user using the Send API.
Param | Type | Description |
---|---|---|
template | Object | Object of the template. |
options | Object | Other optional parameters. For example, messaging types or tags. |
Example:
context.sendTemplate({
templateType: 'button',
text: 'title',
buttons: [
{
type: 'postback',
title: 'Start Chatting',
payload: 'USER_DEFINED_PAYLOAD',
},
],
});
sendButtonTemplate(title, buttons [, options])
- Official Docs
Send button message templates to the user using the Send API.
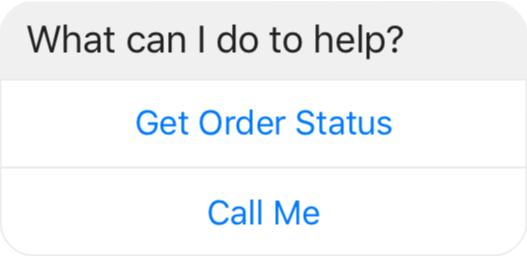
Param | Type | Description |
---|---|---|
title | String | Text that appears above the buttons. |
buttons | Object[] | Array of button. Set of 1-3 buttons that appear as call-to-actions. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
context.sendButtonTemplate('What do you want to do next?', [
{
type: 'web_url',
url: 'https://petersapparel.parseapp.com',
title: 'Show Website',
},
{
type: 'postback',
title: 'Start Chatting',
payload: 'USER_DEFINED_PAYLOAD',
},
]);
sendGenericTemplate(elements [, options])
- Official Docs
Send generic message templates to the user using the Send API.
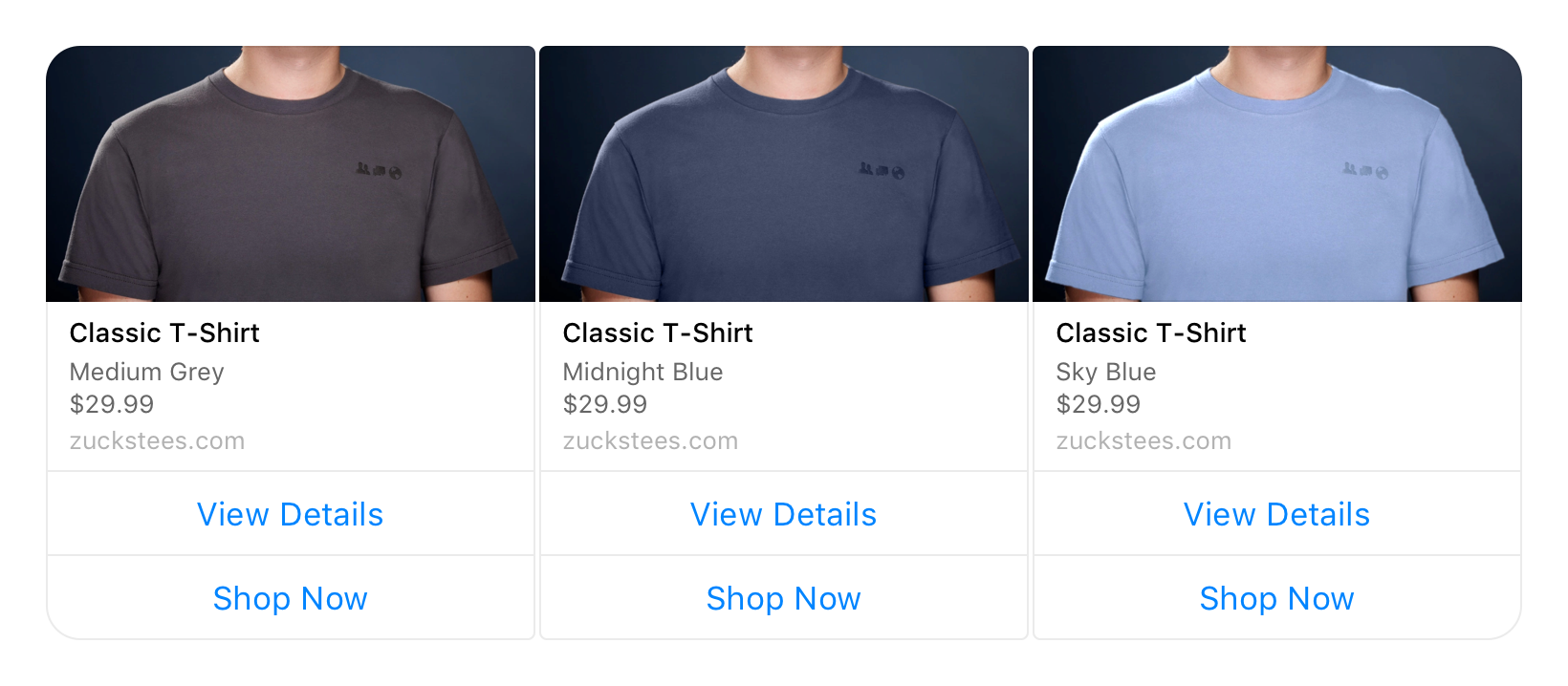
Param | Type | Description |
---|---|---|
elements | Object[] | Array of element. Data for each bubble in message. |
options | Object | Other optional parameters, such as imageAspectRatio , messaging types and tags. |
Example:
context.sendGenericTemplate(
[
{
title: "Welcome to Peter's Hats",
imageUrl: 'https://petersfancybrownhats.com/company_image.png',
subtitle: "We've got the right hat for everyone.",
defaultAction: {
type: 'web_url',
url: 'https://peterssendreceiveapp.ngrok.io/view?item=103',
messengerExtensions: true,
webviewHeightRatio: 'tall',
fallbackUrl: 'https://peterssendreceiveapp.ngrok.io/',
},
buttons: [
{
type: 'postback',
title: 'Start Chatting',
payload: 'DEVELOPER_DEFINED_PAYLOAD',
},
],
},
],
{ imageAspectRatio: 'square' }
);
Adding a tag to a message allows you to send it outside the 24+1 window, for a limited number of use cases, per Messenger Platform policy.
Example:
context.sendGenericTemplate(
[
{
// ...
},
],
{ tag: 'CONFIRMED_EVENT_UPDATE' }
);
Available tags:
CONFIRMED_EVENT_UPDATE
POST_PURCHASE_UPDATE
ACCOUNT_UPDATE
HUMAN_AGENT
sendMediaTemplate(elements [, options])
- Official Docs
Send media message templates to the user using the Send API.
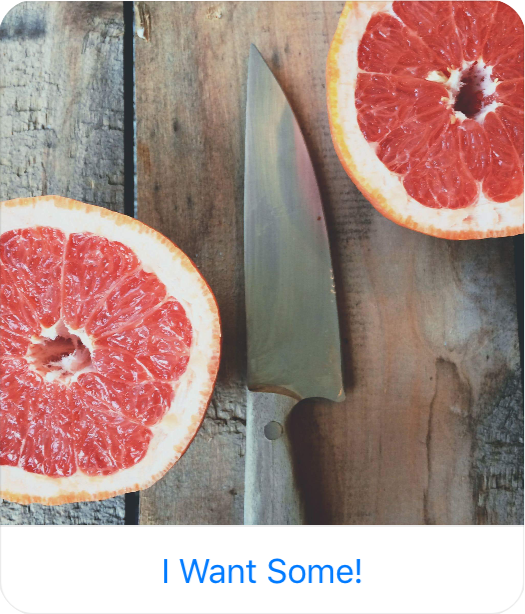
Param | Type | Description |
---|---|---|
elements | Object[] | Array of element. Only one element is allowed. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
context.sendMediaTemplate([
{
mediaType: 'image',
attachmentId: '1854626884821032',
buttons: [
{
type: 'web_url',
url: 'https://en.wikipedia.org/wiki/Rickrolling',
title: 'View Website',
},
],
},
]);
sendReceiptTemplate(receipt [, options])
- Official Docs
Send receipt message templates to the user using the Send API.
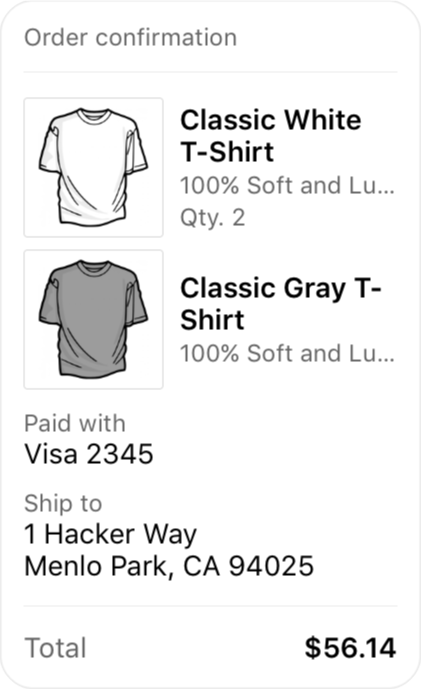
Param | Type | Description |
---|---|---|
receipt | Object | payload of receipt template. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
context.sendReceiptTemplate({
recipientName: 'Stephane Crozatier',
orderNumber: '12345678902',
currency: 'USD',
paymentMethod: 'Visa 2345',
orderUrl: 'http://petersapparel.parseapp.com/order?order_id=123456',
timestamp: '1428444852',
elements: [
{
title: 'Classic White T-Shirt',
subtitle: '100% Soft and Luxurious Cotton',
quantity: 2,
price: 50,
currency: 'USD',
imageUrl: 'http://petersapparel.parseapp.com/img/whiteshirt.png',
},
{
title: 'Classic Gray T-Shirt',
subtitle: '100% Soft and Luxurious Cotton',
quantity: 1,
price: 25,
currency: 'USD',
imageUrl: 'http://petersapparel.parseapp.com/img/grayshirt.png',
},
],
address: {
street1: '1 Hacker Way',
street2: '',
city: 'Menlo Park',
postalCode: '94025',
state: 'CA',
country: 'US',
},
summary: {
subtotal: 75.0,
shippingCost: 4.95,
totalTax: 6.19,
totalCost: 56.14,
},
adjustments: [
{
name: 'New Customer Discount',
amount: 20,
},
{
name: '$10 Off Coupon',
amount: 10,
},
],
});
sendAirlineBoardingPassTemplate(attributes [, options])
- Official Docs
Send airline boarding pass message templates to the user using the Send API.
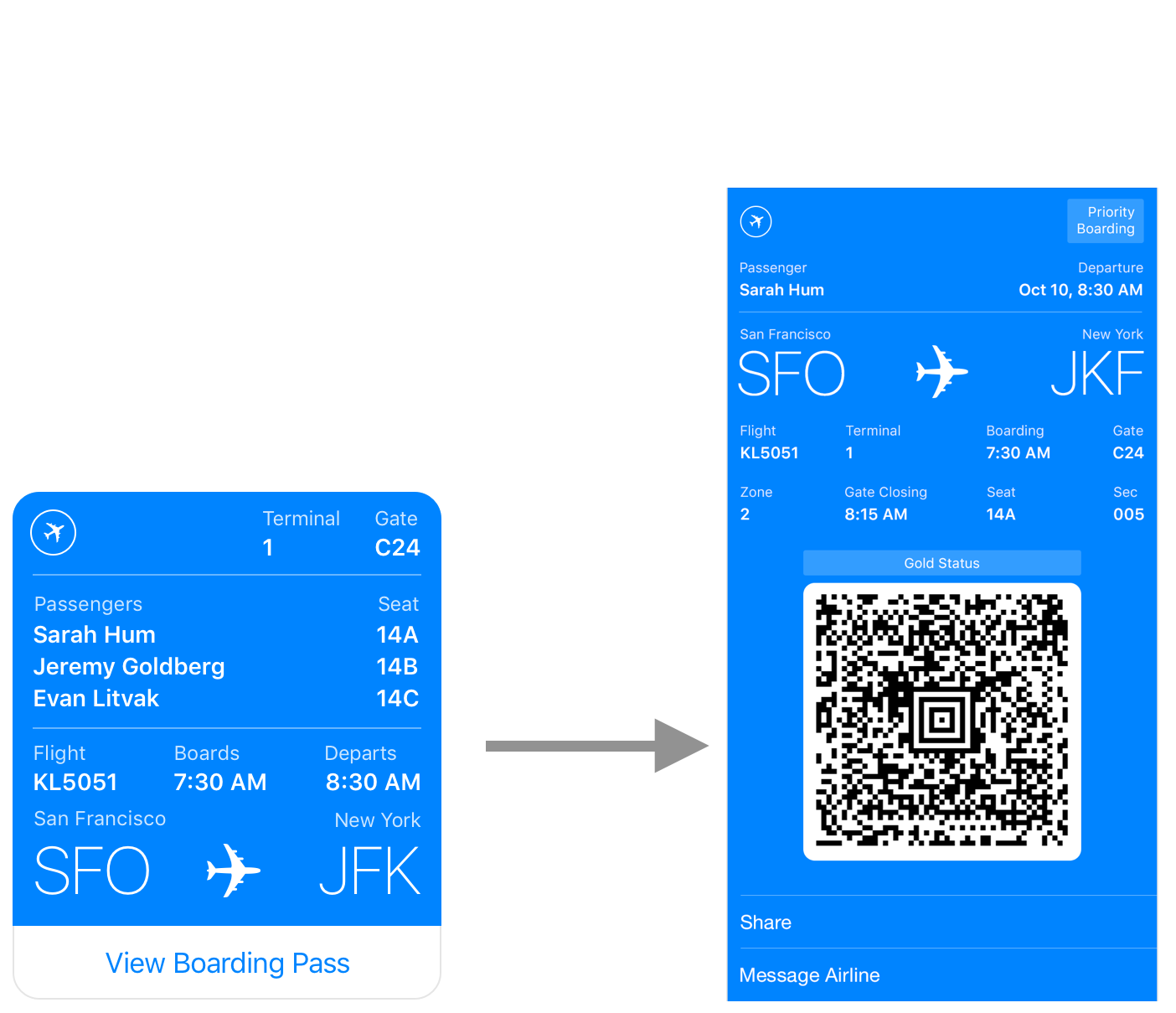
Param | Type | Description |
---|---|---|
attributes | Object | payload of boarding pass template. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
context.sendAirlineBoardingPassTemplate({
introMessage: 'You are checked in.',
locale: 'en_US',
boardingPass: [
{
passengerName: 'SMITH/NICOLAS',
pnrNumber: 'CG4X7U',
travelClass: 'business',
seat: '74J',
auxiliaryFields: [
{
label: 'Terminal',
value: 'T1',
},
{
label: 'Departure',
value: '30OCT 19:05',
},
],
secondaryFields: [
{
label: 'Boarding',
value: '18:30',
},
{
label: 'Gate',
value: 'D57',
},
{
label: 'Seat',
value: '74J',
},
{
label: 'Sec.Nr.',
value: '003',
},
],
logoImageUrl: 'https://www.example.com/en/logo.png',
headerImageUrl: 'https://www.example.com/en/fb/header.png',
qrCode: 'M1SMITH/NICOLAS CG4X7U nawouehgawgnapwi3jfa0wfh',
aboveBarCodeImageUrl: 'https://www.example.com/en/PLAT.png',
flightInfo: {
flightNumber: 'KL0642',
departureAirport: {
airportCode: 'JFK',
city: 'New York',
terminal: 'T1',
gate: 'D57',
},
arrivalAirport: {
airportCode: 'AMS',
city: 'Amsterdam',
},
flightSchedule: {
departureTime: '2016-01-02T19:05',
arrivalTime: '2016-01-05T17:30',
},
},
},
{
passengerName: 'JONES/FARBOUND',
pnrNumber: 'CG4X7U',
travelClass: 'business',
seat: '74K',
auxiliaryFields: [
{
label: 'Terminal',
value: 'T1',
},
{
label: 'Departure',
value: '30OCT 19:05',
},
],
secondaryFields: [
{
label: 'Boarding',
value: '18:30',
},
{
label: 'Gate',
value: 'D57',
},
{
label: 'Seat',
value: '74K',
},
{
label: 'Sec.Nr.',
value: '004',
},
],
logoImageUrl: 'https://www.example.com/en/logo.png',
headerImageUrl: 'https://www.example.com/en/fb/header.png',
qrCode: 'M1JONES/FARBOUND CG4X7U nawouehgawgnapwi3jfa0wfh',
aboveBarCodeImageUrl: 'https://www.example.com/en/PLAT.png',
flightInfo: {
flightNumber: 'KL0642',
departureAirport: {
airportCode: 'JFK',
city: 'New York',
terminal: 'T1',
gate: 'D57',
},
arrivalAirport: {
airportCode: 'AMS',
city: 'Amsterdam',
},
flightSchedule: {
departureTime: '2016-01-02T19:05',
arrivalTime: '2016-01-05T17:30',
},
},
},
],
});
sendAirlineCheckinTemplate(attributes [, options])
- Official Docs
Send airline checkin message templates to the user using the Send API.
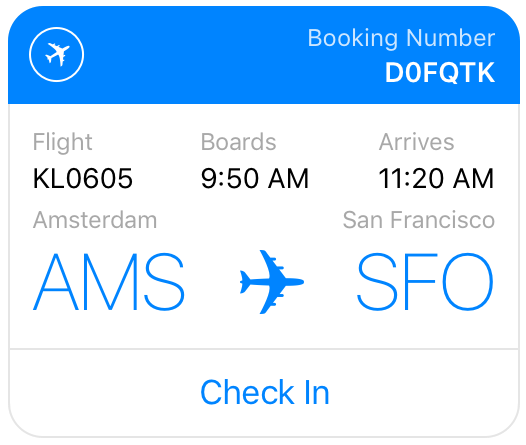
Param | Type | Description |
---|---|---|
attributes | Object | payload of checkin template. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
context.sendAirlineCheckinTemplate({
introMessage: 'Check-in is available now.',
locale: 'en_US',
pnrNumber: 'ABCDEF',
flightInfo: [
{
flightNumber: 'f001',
departureAirport: {
airportCode: 'SFO',
city: 'San Francisco',
terminal: 'T4',
gate: 'G8',
},
arrivalAirport: {
airportCode: 'SEA',
city: 'Seattle',
terminal: 'T4',
gate: 'G8',
},
flightSchedule: {
boardingTime: '2016-01-05T15:05',
departureTime: '2016-01-05T15:45',
arrivalTime: '2016-01-05T17:30',
},
},
],
checkinUrl: 'https://www.airline.com/check-in',
});
sendAirlineItineraryTemplate(attributes [, options])
- Official Docs
Send airline itinerary message templates to the user using the Send API.
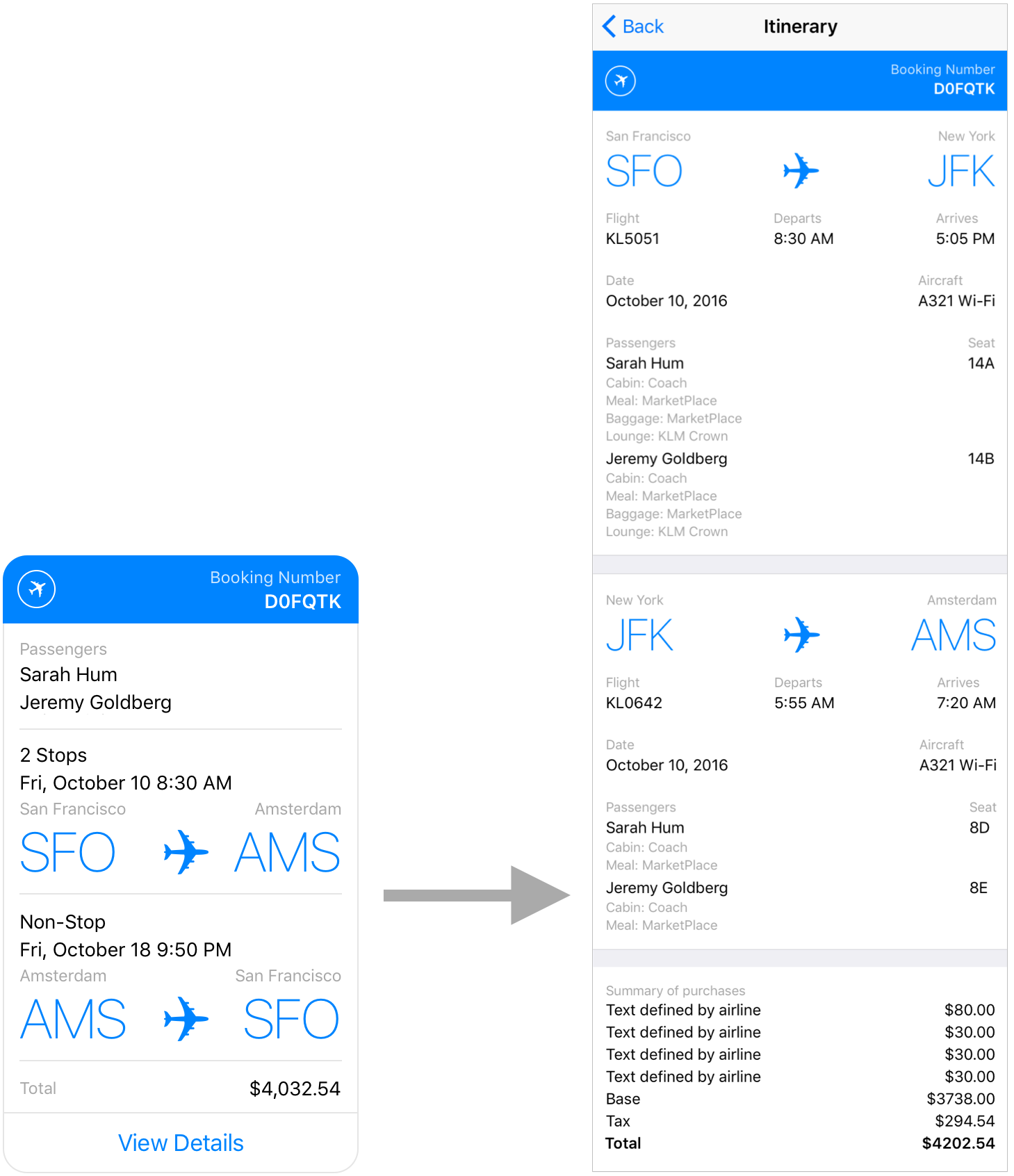
Param | Type | Description |
---|---|---|
attributes | Object | payload of itinerary template. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
context.sendAirlineItineraryTemplate({
introMessage: "Here's your flight itinerary.",
locale: 'en_US',
pnrNumber: 'ABCDEF',
passengerInfo: [
{
name: 'Farbound Smith Jr',
ticketNumber: '0741234567890',
passengerId: 'p001',
},
{
name: 'Nick Jones',
ticketNumber: '0741234567891',
passengerId: 'p002',
},
],
flightInfo: [
{
connectionId: 'c001',
segmentId: 's001',
flightNumber: 'KL9123',
aircraftType: 'Boeing 737',
departureAirport: {
airportCode: 'SFO',
city: 'San Francisco',
terminal: 'T4',
gate: 'G8',
},
arrivalAirport: {
airportCode: 'SLC',
city: 'Salt Lake City',
terminal: 'T4',
gate: 'G8',
},
flightSchedule: {
departureTime: '2016-01-02T19:45',
arrivalTime: '2016-01-02T21:20',
},
travelClass: 'business',
},
{
connectionId: 'c002',
segmentId: 's002',
flightNumber: 'KL321',
aircraftType: 'Boeing 747-200',
travelClass: 'business',
departureAirport: {
airportCode: 'SLC',
city: 'Salt Lake City',
terminal: 'T1',
gate: 'G33',
},
arrivalAirport: {
airportCode: 'AMS',
city: 'Amsterdam',
terminal: 'T1',
gate: 'G33',
},
flightSchedule: {
departureTime: '2016-01-02T22:45',
arrivalTime: '2016-01-03T17:20',
},
},
],
passengerSegmentInfo: [
{
segmentId: 's001',
passengerId: 'p001',
seat: '12A',
seatType: 'Business',
},
{
segmentId: 's001',
passengerId: 'p002',
seat: '12B',
seatType: 'Business',
},
{
segmentId: 's002',
passengerId: 'p001',
seat: '73A',
seatType: 'World Business',
productInfo: [
{
title: 'Lounge',
value: 'Complimentary lounge access',
},
{
title: 'Baggage',
value: '1 extra bag 50lbs',
},
],
},
{
segmentId: 's002',
passengerId: 'p002',
seat: '73B',
seatType: 'World Business',
productInfo: [
{
title: 'Lounge',
value: 'Complimentary lounge access',
},
{
title: 'Baggage',
value: '1 extra bag 50lbs',
},
],
},
],
priceInfo: [
{
title: 'Fuel surcharge',
amount: '1597',
currency: 'USD',
},
],
basePrice: '12206',
tax: '200',
totalPrice: '14003',
currency: 'USD',
});
sendAirlineFlightUpdateTemplate(attributes [, options])
- Official Docs
Send airline flight update message templates to the user using the Send API.
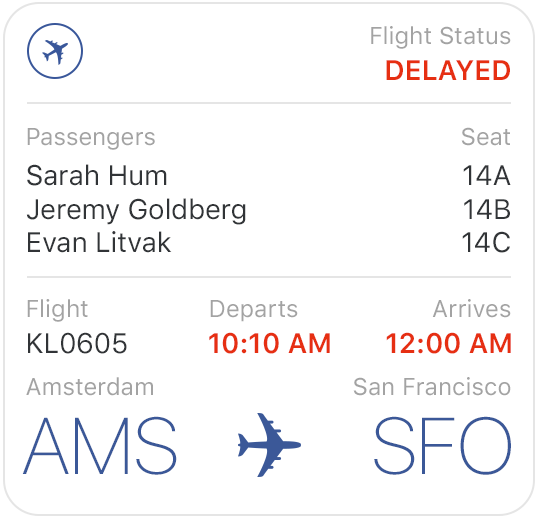
Param | Type | Description |
---|---|---|
attributes | Object | payload of update template. |
options | Object | Other optional parameters. For example, messaging types. |
Example:
context.sendAirlineFlightUpdateTemplate({
introMessage: 'Your flight is delayed',
updateType: 'delay',
locale: 'en_US',
pnrNumber: 'CF23G2',
updateFlightInfo: {
flightNumber: 'KL123',
departureAirport: {
airportCode: 'SFO',
city: 'San Francisco',
terminal: 'T4',
gate: 'G8',
},
arrivalAirport: {
airportCode: 'AMS',
city: 'Amsterdam',
terminal: 'T4',
gate: 'G8',
},
flightSchedule: {
boardingTime: '2015-12-26T10:30',
departureTime: '2015-12-26T11:30',
arrivalTime: '2015-12-27T07:30',
},
},
});
Quick Replies - Official Docs
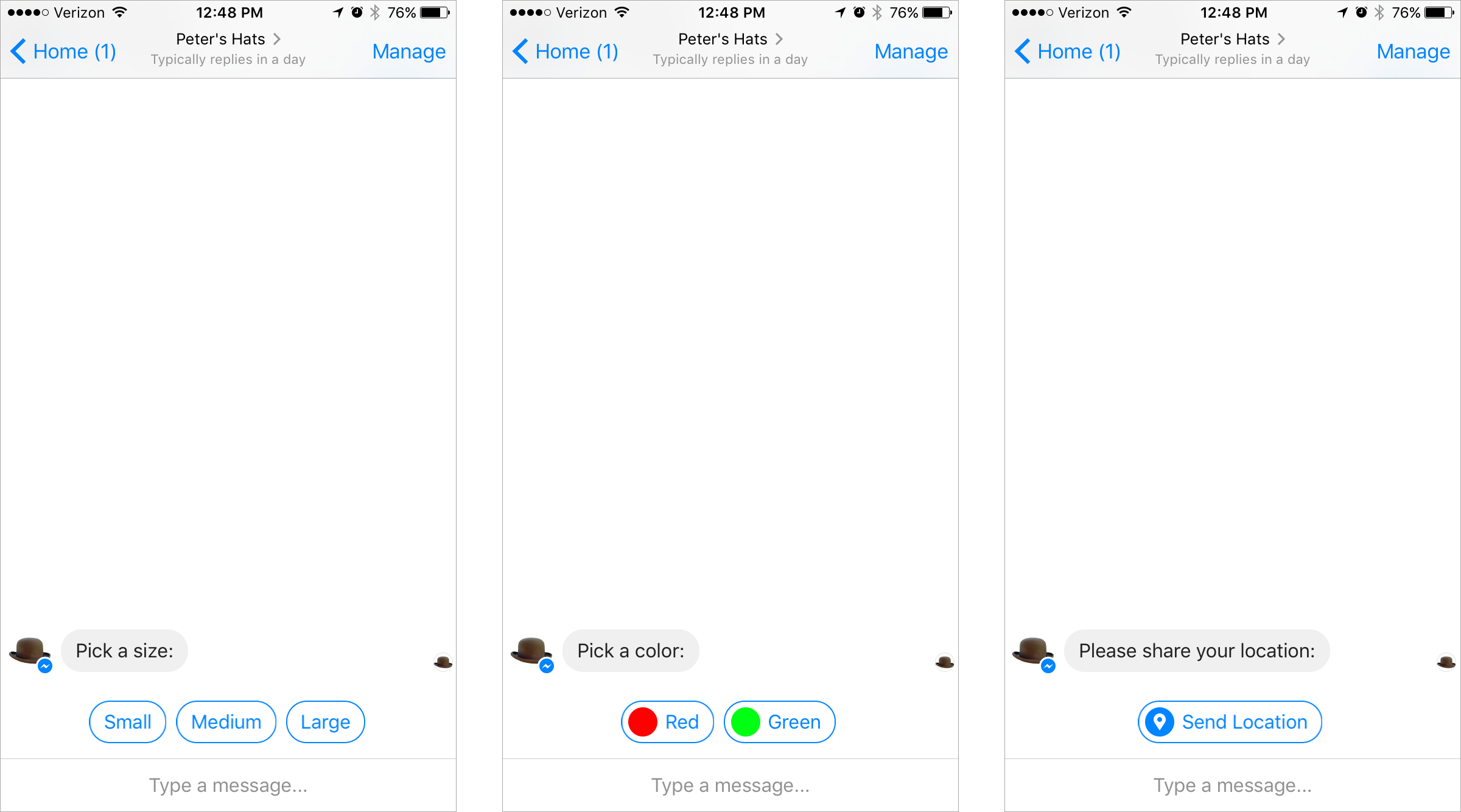
To send messages with quick replies to the user using the Send API, pass quickReplies
option to send message methods, for example, with sendText
:
context.sendText('Pick a color:', {
quickReplies: [
{
contentType: 'text',
title: 'Red',
payload: 'DEVELOPER_DEFINED_PAYLOAD_FOR_PICKING_RED',
},
],
});
with sendImage
:
context.sendImage('https://example.com/vr.jpg', {
quickReplies: [
{
contentType: 'text',
title: 'Red',
payload: 'DEVELOPER_DEFINED_PAYLOAD_FOR_PICKING_RED',
},
],
});
It works with all of send message methods.
Sender Actions - Official Docs
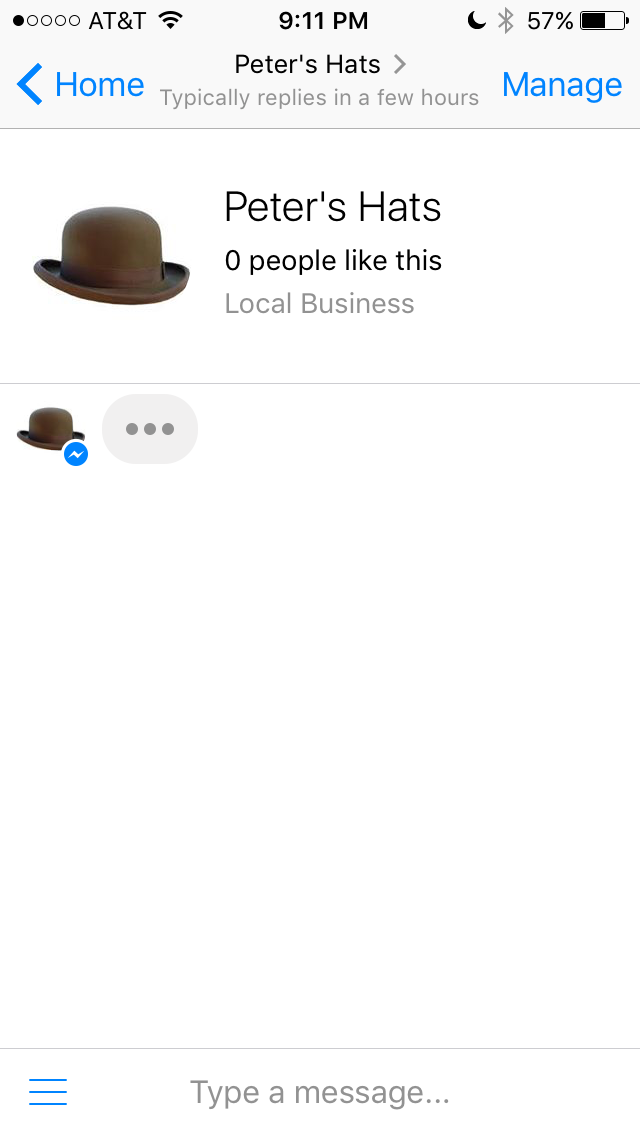
sendSenderAction(action)
Send sender actions to the user using the Send API, to let users know you are processing their request.
Param | Type | Description |
---|---|---|
action | String | Name of the action. |
Example:
context.sendSenderAction('typing_on');
markSeen()
Mark last message as read for the user.
Example:
context.markSeen();
typingOn()
Turn typing indicators on for the user.
Example:
context.typingOn();
typingOff()
Turn typing indicators off for the user.
Example:
context.typingOff();
Targeting Broadcast Messages - Official Docs
associateLabel(labelId)
Associating a Label to the user
Param | Type | Description |
---|---|---|
labelId | String | ID of the custom label. |
Example:
context.associateLabel(LABEL_ID);
dissociateLabel(labelId)
Removing a Label from the user.
Param | Type | Description |
---|---|---|
labelId | String | ID of the custom label. |
Example:
context.dissociateLabel(LABEL_ID);
getAssociatedLabels()
Retrieving Labels Associated with the user.
Example:
context.getAssociatedLabels().then((result) => {
console.log(result);
// {
// data: [
// {
// name: 'myLabel',
// id: '1001200005003',
// },
// {
// name: 'myOtherLabel',
// id: '1001200005002',
// },
// ],
// paging: {
// cursors: {
// before:
// 'QVFIUmx1WTBpMGpJWXprYzVYaVhabW55dVpycko4U2xURGE5ODNtNFZAPal94a1hTUnNVMUtoMVVoTzlzSDktUkMtQkUzWEFLSXlMS3ZALYUw3TURLelZAPOGVR',
// after:
// 'QVFIUmItNkpTbjVzakxFWGRydzdaVUFNNnNPaUl0SmwzVHN5ZAWZAEQ3lZANDAzTXFIM0NHbHdYSkQ5OG1GaEozdjkzRmxpUFhxTDl4ZAlBibnE4LWt1eGlTa3Bn',
// },
// },
// }
});
User Profile API - Official Docs
getUserProfile()
Retrieving profile of the user.
Example:
context.getUserProfile().then((user) => {
console.log(user);
// {
// firstName: 'Johnathan',
// lastName: 'Jackson',
// profilePic: 'https://example.com/pic.png',
// locale: 'en_US',
// timezone: 8,
// gender: 'male',
// }
});
Handover Protocol API
passThreadControl(targetAppId [, metadata])
- Official Docs
Passes control of the thread from your app to another app.
Param | Type | Description |
---|---|---|
targetAppId | Number | The app ID of the Secondary Receiver to pass thread control to. |
metadata | String | Metadata passed to the receiving app in the pass_thread_control webhook event. |
Example:
context.passThreadControl(APP_ID);
passThreadControlToPageInbox(metadata)
- Official Docs
Passes control of the thread from your app to "Page Inbox" app.
Param | Type | Description |
---|---|---|
metadata | String | Metadata passed to the receiving app in the pass_thread_control webhook event. |
Example:
context.passThreadControlToPageInbox();
takeThreadControl(metadata)
- Official Docs
Takes control of the thread from a Secondary Receiver app.
Param | Type | Description |
---|---|---|
metadata | String | Metadata passed back to the secondary app in the take_thread_control webhook event. |
Example:
context.takeThreadControl();
requestThreadControl(metadata)
- Official Docs
Requests control of the thread from a Primary Receiver app.
Param | Type | Description |
---|---|---|
metadata | String | Metadata passed to the primary app in the request_thread_control webhook event. |
Example:
context.requestThreadControl();