ViberContext
Send API
sendMessage(message)
- Official Docs
Sending a message to the user.
Param | Type | Description |
---|---|---|
message | Object | Message and options to be sent. |
Example:
context.sendMessage({
type: 'text',
text: 'Hello',
});
Note: Maximum total JSON size of the request is 30kb.
sendText(text [, options])
- Official Docs
Sending a text message to the user.

Param | Type | Description |
---|---|---|
text | String | The text of the message. |
options | Object | Other optional parameters. |
Example:
context.sendText('Hello');
sendPicture(picture [, options])
- Official Docs
Sending a picture message to the user.
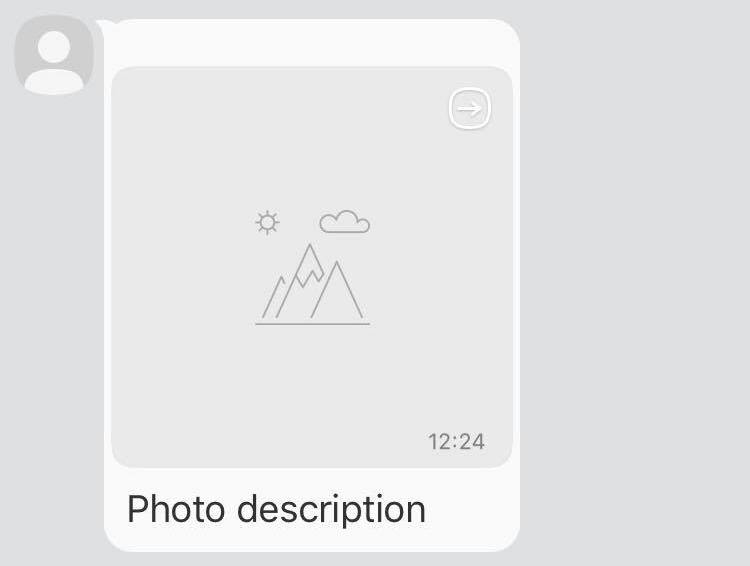
Param | Type | Description |
---|---|---|
picture | Object | |
picture.text | String | Description of the photo. Can be an empty string if irrelevant. Max 120 characters. |
picture.media | String | URL of the image (JPEG). Max size 1 MB. Only JPEG format is supported. Other image formats as well as animated GIFs can be sent as URL messages or file messages. |
picture.thumbnail | String | URL of a reduced size image (JPEG). Max size 100 kb. Recommended: 400x400. Only JPEG format is supported. |
options | Object | Other optional parameters. |
Example:
context.sendPicture({
text: 'Photo description',
media: 'http://www.images.com/img.jpg',
thumbnail: 'http://www.images.com/thumb.jpg',
});
sendVideo(video [, options])
- Official Docs
Sending a video message to the user.
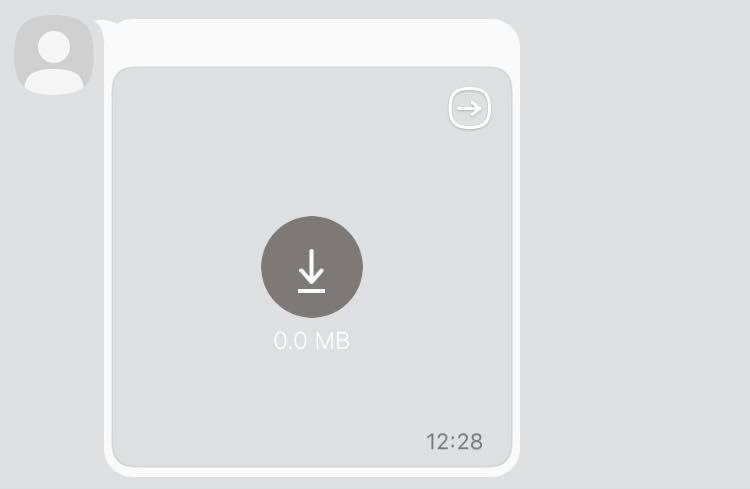
Param | Type | Description |
---|---|---|
video | Object | |
video.media | String | URL of the video (MP4, H264). Max size 50 MB. Only MP4 and H264 are supported. |
video.size | Number | Size of the video in bytes. |
video.duration | Number | Video duration in seconds; will be displayed to the receiver. Max 180 seconds. |
video.thumbnail | String | URL of a reduced size image (JPEG). Max size 100 kb. Recommended: 400x400. Only JPEG format is supported. |
options | Object | Other optional parameters. |
Example:
context.sendVideo({
media: 'http://www.images.com/video.mp4',
size: 10000,
thumbnail: 'http://www.images.com/thumb.jpg',
duration: 10,
});
sendFile(file [, options])
- Official Docs
Sending a file message to the user.
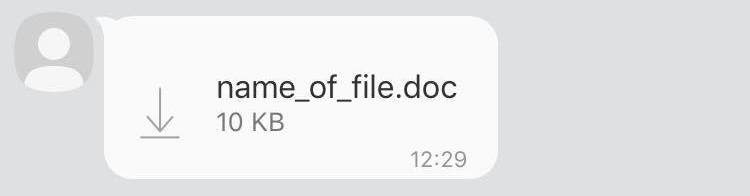
Param | Type | Description |
---|---|---|
file | Object | |
file.media | String | URL of the file. Max size 50 MB. See forbidden file formats for unsupported file types. |
file.size | Number | Size of the file in bytes. |
file.file_name | String | Name of the file. File name should include extension. Max 256 characters (including file extension). |
options | Object | Other optional parameters. |
Example:
context.sendFile({
media: 'http://www.images.com/file.doc',
size: 10000,
file_name: 'name_of_file.doc',
});
sendContact(contact [, options])
- Official Docs
Sending a contact message to the user.
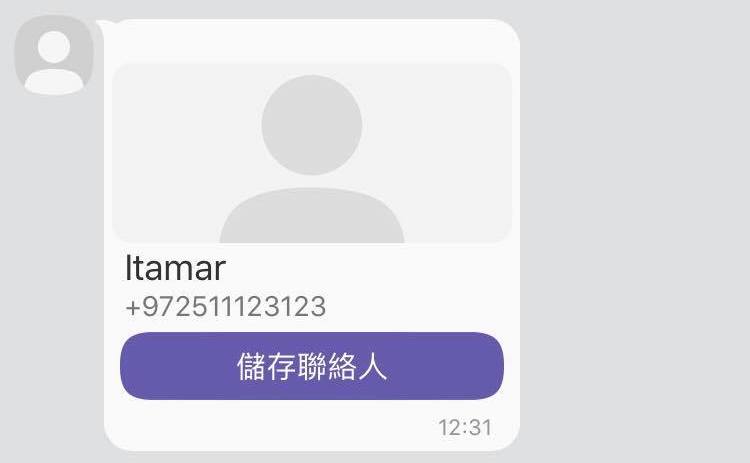
Param | Type | Description |
---|---|---|
contact | Object | |
contact.name | String | Name of the contact. Max 28 characters. |
contact.phone_number | String | Phone number of the contact. Max 18 characters. |
options | Object | Other optional parameters. |
Example:
context.sendContact({
name: 'Itamar',
phone_number: '+972511123123',
});
sendLocation(location [, options])
- Official Docs
Sending a location message to the user.
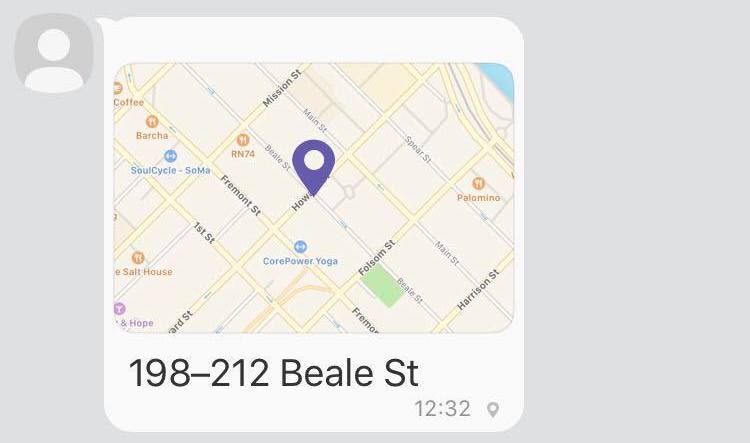
Param | Type | Description |
---|---|---|
location | Object | |
location.lat | String | Latitude (±90°) within valid ranges. |
location.lon | String | Longitude (±180°) within valid ranges. |
options | Object | Other optional parameters. |
Example:
context.sendLocation({
lat: '37.7898',
lon: '-122.3942',
});
sendURL(url [, options])
- Official Docs
Sending an URL message to the user.
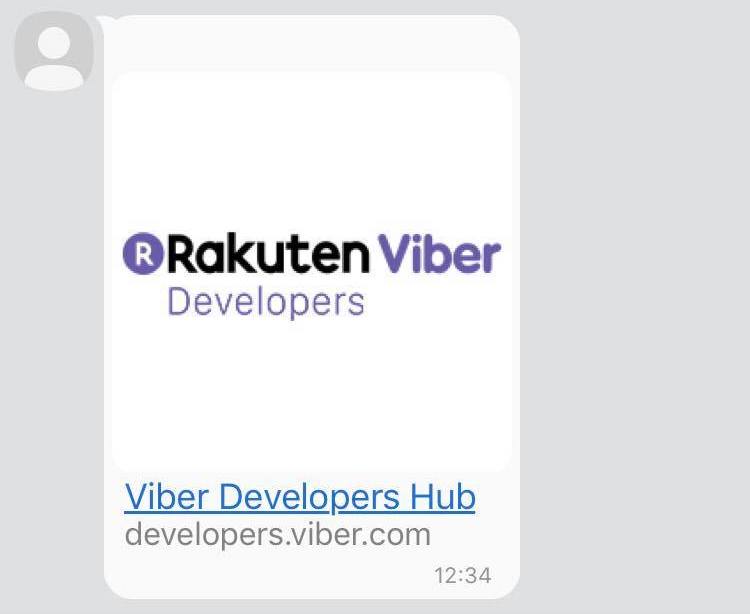
Param | Type | Description |
---|---|---|
url | String | URL. Max 2,000 characters. |
options | Object | Other optional parameters. |
Example:
context.sendURL('http://developers.viber.com');
sendSticker(stickerId [, options])
- Official Docs
Sending a sticker message to the user.
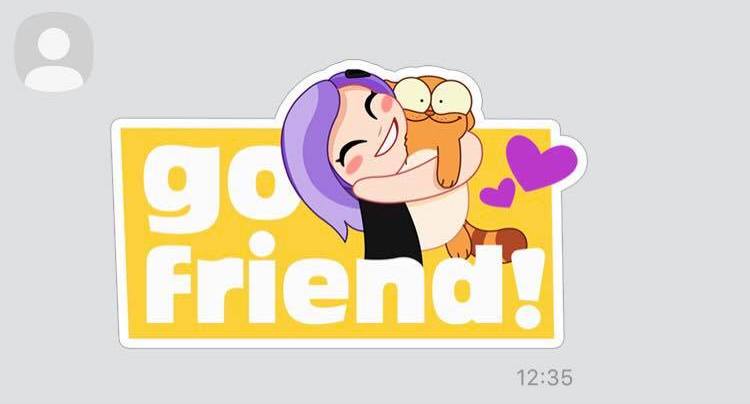
Param | Type | Description |
---|---|---|
stickerId | Number | Unique Viber sticker ID. For examples visit the sticker IDs page. |
options | Object | Other optional parameters. |
Example:
context.sendSticker(46105);
sendCarouselContent(richMedia [, options])
- Official Docs
Sending a carousel content message to the user.
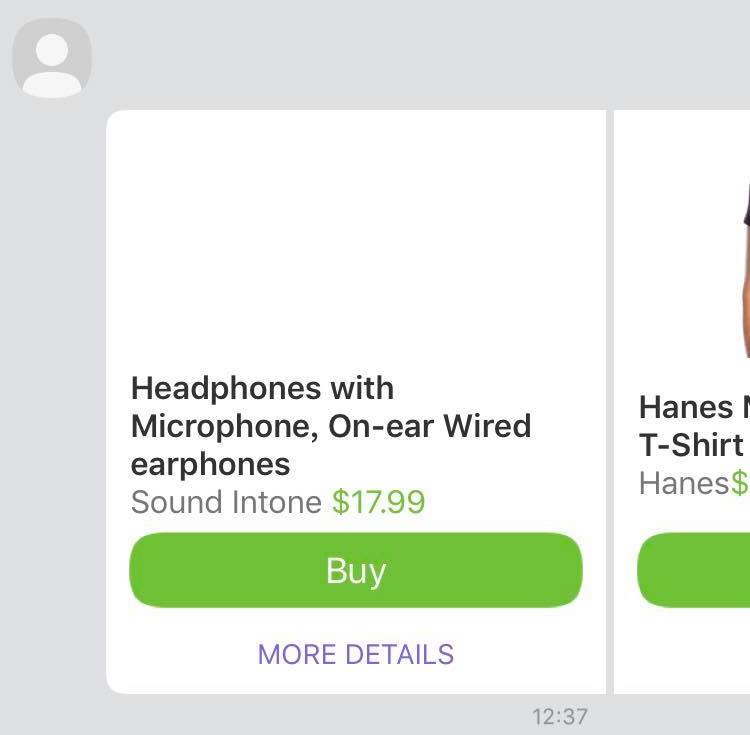
Param | Type | Description |
---|---|---|
richMedia.ButtonsGroupColumns | Number | Number of columns per carousel content block. Default 6 columns. Possible values: 1 - 6. |
richMedia.ButtonsGroupRows | Number | Number of rows per carousel content block. Default 7 rows. Possible values: 1 - 7. |
richMedia.Buttons | Array<Object> | Array of buttons. Max of 6 ButtonsGroupColumns ButtonsGroupRows. |
options | Object | Other optional parameters. |
Example:
context.sendCarouselContent({
Type: 'rich_media',
ButtonsGroupColumns: 6,
ButtonsGroupRows: 7,
BgColor: '#FFFFFF',
Buttons: [
{
Columns: 6,
Rows: 3,
ActionType: 'open-url',
ActionBody: 'https://www.google.com',
Image: 'http://html-test:8080/myweb/guy/assets/imageRMsmall2.png',
},
{
Columns: 6,
Rows: 2,
Text: '<font color=#323232><b>Headphones with Microphone, On-ear Wired earphones</b></font><font color=#777777><br/>Sound Intone </font><font color=#6fc133>$17.99</font>',
ActionType: 'open-url',
ActionBody: 'https://www.google.com',
TextSize: 'medium',
TextVAlign: 'middle',
TextHAlign: 'left',
},
{
Columns: 6,
Rows: 1,
ActionType: 'reply',
ActionBody: 'https://www.google.com',
Text: '<font color=#ffffff>Buy</font>',
TextSize: 'large',
TextVAlign: 'middle',
TextHAlign: 'middle',
Image: 'https://s14.postimg.org/4mmt4rw1t/Button.png',
},
{
Columns: 6,
Rows: 1,
ActionType: 'reply',
ActionBody: 'https://www.google.com',
Text: '<font color=#8367db>MORE DETAILS</font>',
TextSize: 'small',
TextVAlign: 'middle',
TextHAlign: 'middle',
},
{
Columns: 6,
Rows: 3,
ActionType: 'open-url',
ActionBody: 'https://www.google.com',
Image: 'https://s16.postimg.org/wi8jx20wl/image_RMsmall2.png',
},
{
Columns: 6,
Rows: 2,
Text: "<font color=#323232><b>Hanes Men's Humor Graphic T-Shirt</b></font><font color=#777777><br/>Hanes</font><font color=#6fc133>$10.99</font>",
ActionType: 'open-url',
ActionBody: 'https://www.google.com',
TextSize: 'medium',
TextVAlign: 'middle',
TextHAlign: 'left',
},
{
Columns: 6,
Rows: 1,
ActionType: 'reply',
ActionBody: 'https://www.google.com',
Text: '<font color=#ffffff>Buy</font>',
TextSize: 'large',
TextVAlign: 'middle',
TextHAlign: 'middle',
Image: 'https://s14.postimg.org/4mmt4rw1t/Button.png',
},
{
Columns: 6,
Rows: 1,
ActionType: 'reply',
ActionBody: 'https://www.google.com',
Text: '<font color=#8367db>MORE DETAILS</font>',
TextSize: 'small',
TextVAlign: 'middle',
TextHAlign: 'middle',
},
],
});
Keyboards - Official Docs
The Viber API allows sending a custom keyboard using the send_message API, to supply the user with a set of predefined replies or actions. Keyboards can be attached to any message type and be sent and displayed together. To attach a keyboard to a message simply add the keyboard’s parameters to the options:
context.sendText('Hello', {
keyboard: {
DefaultHeight: true,
BgColor: '#FFFFFF',
Buttons: [
{
Columns: 6,
Rows: 1,
BgColor: '#2db9b9',
BgMediaType: 'gif',
BgMedia: 'http://www.url.by/test.gif',
BgLoop: true,
ActionType: 'open-url',
ActionBody: 'www.tut.by',
Image: 'www.tut.by/img.jpg',
Text: 'Key text',
TextVAlign: 'middle',
TextHAlign: 'center',
TextOpacity: 60,
TextSize: 'regular',
},
],
},
});
Which in turn will look like this:
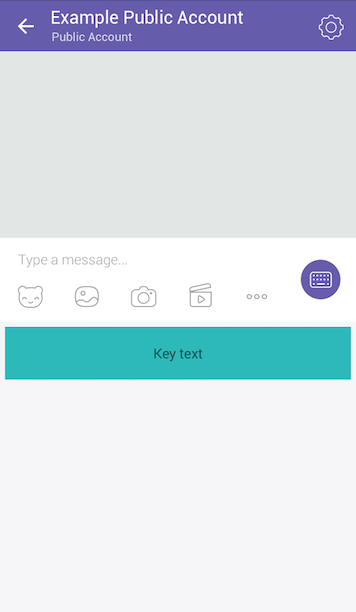
Get User Details
getUserDetails()
- Official Docs
It will fetch the details of the user.
Example:
context.getUserDetails().then((user) => {
console.log(user);
// {
// id: '01234567890A=',
// name: 'John McClane',
// avatar: 'http://avatar.example.com',
// country: 'UK',
// language: 'en',
// primary_device_os: 'android 7.1',
// api_version: 1,
// viber_version: '6.5.0',
// mcc: 1,
// mnc: 1,
// device_type: 'iPhone9,4',
// };
});
-->
Get Online
getOnlineStatus()
- Official Docs
It will fetch the online status of the user.
Example:
context.getOnlineStatus().then((status) => {
console.log(status);
// {
// id: '01234567891=',
// online_status: 1,
// online_status_message: 'offline',
// last_online: 1457764197627,
// }
});